Python Try Except is a construct used in Python to handle exceptions gracefully without crashing.
Exception handling makes your program code more reliable and less likely to fail. This article guides handling exceptions and the common scenarios in which exception handling is ideal. As a bonus, we will also cover how to raise exceptions.
What Is Exception Handling?
Exceptions are critical anomalies and errors that arise during a program’s execution. If not handled, exceptions will crash the program. Therefore, exception handling is a way to handle exceptions to ensure they do not crash the program.
Here’s an example to illustrate what an exception is.
user_input = input("Enter a number: ")
num = int(user_input)
print("Your number doubled is:", num * 2)
At first glance, it appears there’s nothing wrong with the above program. It receives input from the user and converts it to an integer. Next, it displays the integer provided by the user doubled.
The program runs fine if you run it with an input value of 5. See below.

But suppose you ran the same program again. Only this time, instead of using 5 as your input, you enter the string “hello”. The program will crash. The string “hello” cannot be converted to an integer, so an exception is raised, and the program crashes.

Why Are Exceptions Raised and Why Should You Handle Them?
Exceptions are raised because we often decompose programs into functions when coding them. These functions are then called to perform different tasks.
In the example above, we called the input function to receive the user’s input, then we called the int function to convert the string input to an integer, and lastly, we called the print function to display some output.
However, as functions carry out their actions, they may encounter errors they do not know how to handle. In this case, the said functions must stop running and communicate that an error has been encountered. To communicate, they will raise exceptions.
The code that is called the function is responsible for listening to those exceptions and reacting appropriately. If this is not done, the program will crash after encountering errors, as we saw in the earlier example.
Therefore, exceptions are essentially a communication mechanism that enables a function that has been called to send a distress signal to the code that called it. And the reacting appropriately alluded to earlier is the essence of exception handling.
Different Kinds of Exceptions
It is important to know that not all exceptions are the same. There are different kinds of exceptions raised for different errors encountered. For example, if you attempt to divide a number by zero, a ZeroDivisionError is raised. And a TypeError is raised when you attempt an operation with an invalid data type. Here’s a complete list of types of exceptions.
How to Handle Exceptions
As explained earlier, exceptions are distress signals made by functions we call. Our code should, therefore, listen to these distress signals and react appropriately when they are sounded. To handle exceptions appropriately, we use Python Try Except constructs. The basic structure for the construct is as follows:
try:
# Code to try and run
except:
# Code to run if an exception is raised
finally:
# Code to run in the end, whether or not an exception is raised
As you can see, the construct is made up of three keywords that are explained below:
try
The try
keyword marks the beginning of a Python Try Except construct. In addition, it marks a block of code that could potentially raise an exception. It is an instruction to the Python interpreter to try to run code in the block. If an exception is raised, the program stops immediately and jumps to execute the code written inside the except block.
except
The except
keyword marks the block of code that will be executed if an exception is raised while executing the try block. You can define multiple except blocks for different types of exceptions that could be raised. This will be illustrated later.
finally
The finally
keyword is the third and last keyword used in Python Try Except. It marks a block of code that will be executed whether or not an exception is raised.
An Example
Here’s an example of how the keywords above can handle an exception. We will modify the previous example to this.
try:
user_input = input("Enter a number: ")
num = int(user_input)
print("Your number doubled is:", num * 2)
except:
print("Something went wrong")
finally:
print("This code will be executed no matter what")
If you run the above code with 5, a valid input, as your input, you will get the following:

And if you run it with “hello” as your input, you will get the following:
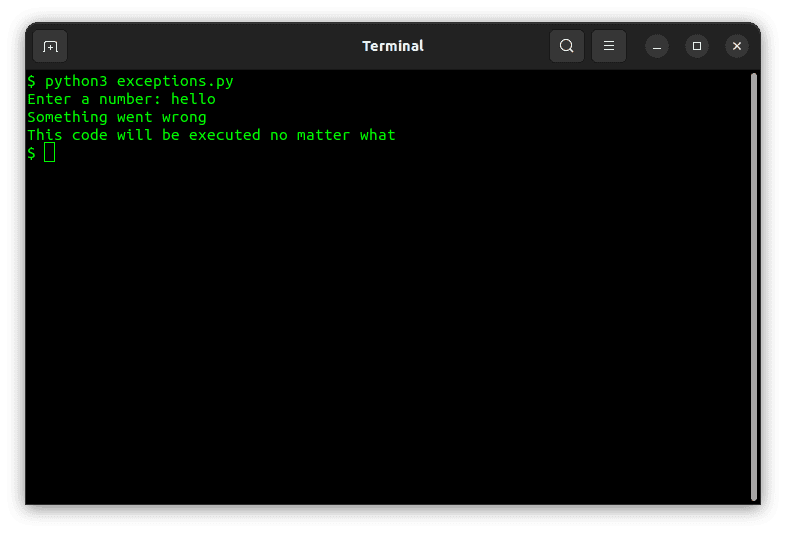
So when no exception was raised while executing code in the try block, the computer moved on to the finally
block. However, when an exception was raised while executing code in the try block, the computer moved to the except block, and then the finally
block.
You can also handle exceptions for specific kinds of errors. For example, if you want to handle ValueError
and KeyboardInterrupt
exceptions in a specific way, you could modify the above code like so:
try:
user_input = input("Enter a number: ")
num = int(user_input)
print("Your number doubled is:", num * 2)
except ValueError:
print("Value can't be converted to int")
except KeyboardInterrupt:
print("Received a keyboard interrupt")
except:
print("Catch-all exception block")
finally:
print("This code will be executed no matter what")
In the code above, we have 3 except
blocks. The first except
block catches only ValueError
exceptions, while the second only catches KeyboardInterrupt
exceptions. The last except
block does not have an associated exception type to listen for. As a result, it catches the remainder of exceptions not caught by the first two blocks.
Running the code above, you should get an output similar to this:


When an exception is raised, you can get more information about the exception in the exception object. To get access to the exception object, you use the as
keyword. It is used as follows:
try:
user_input = input("Enter a number: ")
num = int(user_input)
print("Your number doubled is:", num * 2)
except ValueError as e:
print("Value Error:", e)
except KeyboardInterrupt as e:
print("Keyboard Interrupt:", e)
except Exception as e:
print("Some other exception", e)
How to Raise Exceptions
Until now, we have been dealing with exceptions raised by other functions. However, it is also possible for you to raise exceptions in your code. To raise an exception, we use the raise keyword. We also specify a class that represents what type of exception we want to raise and the human-readable message associated with the exception.
We use the Exception class in the following example to raise a generic exception. Next, we pass the message to the class’ constructor.
raise Exception('Something went wrong')
If you run the snippet above as a program, you’ll get output similar to this:

You can also specify different kinds of exceptions. For example, you can raise a TypeError exception when a value has the wrong data type:
def double(x):
if isinstance(x, int):
return x * 2
else
raise TypeError('x should be an int')
Or if the value specified is outside the acceptable bounds, you can raise a ValueError:
def say_hello(name):
if name == '':
raise ValueError('Value outside bounds')
else:
print('Hello', name)
You can also create your exception types by subclassing the Exception class. Here is an example:
class InvalidHTTPMethod(Exception):
pass
In the example above, we created a class InvalidHTTPMethod, which inherits from the Exception class. We can use it in the same way as before to raise exceptions:
raise InvalidHTTPMethod('Must be GET or POST')
Common Use Cases for Exception Handling
Exception Handling is used in a lot of scenarios. The previous example showed how it can handle exceptions due to user input. This section will cover two additional situations where exception handling is useful. These are handling exceptions as a result of failed network requests and handling exceptions while reading files.
Making Network Requests
In the example below, we are making a request to Google. We are listening for exceptions to handle them. These exceptions are defined in the requests.exceptions object.
import requests
try:
response = requests.get("https://google.com")
# Check if the response status code is in the 200-299 range (successful response)
if 200 <= response.status_code < 300:
print("Request was successful!")
else:
print(f"Request failed with status code: {response.status_code}")
except requests.exceptions.RequestException as e:
print(f"RequestException occurred: {e}")
except requests.exceptions.ConnectionError as e:
print(f"ConnectionError occurred: {e}")
except requests.exceptions.Timeout as e:
print(f"Timeout occurred: {e}")
except requests.exceptions.TooManyRedirects as e:
print(f"TooManyRedirects occurred: {e}")
except requests.exceptions.HTTPError as e:
print(f"HTTPError occurred: {e}")
except Exception as e:
print(f"An unexpected error occurred: {e}")
Reading Data From a File
In this last example, we are reading data from the hello.txt file. We are also handling common exceptions that may be raised, such as the FileNotFound error and IOError.
try:
with open(file_path, 'r') as file:
data = file.read()
print("File contents:")
print(data)
except FileNotFoundError as e:
print(f"FileNotFoundError occurred: {e}")
except IOError as e:
print(f"IOError occurred: {e}")
except Exception as e:
print(f"An unexpected error occurred: {e}")
Conclusion
This article explored what exceptions were and why they were raised. We also established that we handle them to make code more reliable and prevent crashing. Lastly, we covered how to handle exceptions as well as how to raise some exceptions.
Next, check out common Python error types and how to resolve them.
-
Full stack web developer and technical writer. Currently learning AI.
-
Rashmi has over 7 years of expertise in content management, SEO, and data research, making her a highly experienced professional. She has a solid academic background and has done her bachelor’s and master’s degree in computer applications…. read more