TypeScript is a superset of JavaScript that helps you write type-safe code.
With TypeScript, you will be able to catch bugs earlier and fix them. One of the important TypeScript features is Enums. An Enum is a popular construct in TypeScript that helps you write safer code.
In this article, I will explain Enums, how to create and use them, and many other things to consider.
What is an Enum?
Enum is short for enumerated type. An enumerated data type is defined by the different values a variable of that type could take. The different values it could take are often called members or elements.
In this article, we will call them members. Enums are not just a TypeScript feature. Rather, enumerated types are also present and used in other programming languages. Using enums enables better type safety in TypeScript programs.
Also read: Top TypeScript Libraries and Runtime to Know as a Developer
Why Use Enums?
Enums help you specify what values a variable could hold. They also help you specify what values a function argument can take. This helps ensure that the developers always provide expected values to variables and function arguments. This will help eliminate bugs and help you write safer and more robust code.
Prerequisites to Work With Enums
In this tutorial, I will demonstrate how to work with enums in TypeScript. To follow along with this tutorial, you need to be able to run and execute TypeScript code.
Therefore you need to have NodeJs installed to execute your JavaScript code. If you do not have TypeScript installed, the video below is a guide on how to do it.
Alternatively, if you want to compile and execute a script immediately, use ts-node
. While that is what I will be doing in this article, for larger projects, I would recommend installing TypeScript. To execute a script using ts-node
, you use the following command:
npx ts-node <scriptname>
This article also assumes you are familiar with JavaScript and basic TypeScript.
Working With a Simple Enum
How to Create a Simple Enum
Enums in TypeScript are created using the enum keyword. The enum’s name follows, and then a list of members that the enum holds. Here’s an example where we declare an enum for the four cardinal points on the compass.
enum Direction {
North,
East,
South,
West
}
Note that there are no quotes around the members in the enum – they are not strings.
How to Use a Simple Enum
You assign an enumerated value to a variable as follows:
const heading: Direction = Direction.North;
Because we are assigning the variable upon declaration, TypeScript can infer the data type. As a result, we do not need to assert the type. Instead, we can write this:
const heading = Direction.North;
To find what value the heading variable has, we can console.log it.
console.log(heading);
The result of this will be:
0

The heading variable contains the value zero even though we assigned the value of Direction.North
to it. This is because each member is assigned a numerical value when enums are created. The first member is assigned 0, the second 1, and so on. Here’s a demonstration:
console.log(Direction.North, Direction.East, Direction.South, Direction.West)
Therefore, you can check if the heading variable contains North by asserting that it equals zero. For example:
// Asserting equality
let isNorth = heading == 0;
// Printing the result
console.log(isNorth);

As demonstrated earlier, Direction.North
is equal to 0. So, instead of checking if heading
is equal to zero, we can check if it is equal to Direction.North
.
// Checking if direction is North
isNorth = heading == Direction.North;
// Printing the result
console.log(isNorth);

All this is useful; chances are that is how you will use enumerated values. However, you can do more, as you will see later. This next section will discuss how you can work with custom values.
Working With Custom Values
When you create an enum, TypeScript automatically assigns numerical values to the members starting from zero. However, you may want your members to have custom values instead of the default ones, in which case, you can assign them. Like so:
enum StarRating {
VeryPoor = 1,
Poor = 2,
Average = 3,
Good = 4,
Excellent = 5
}
Alternatively, you can do the following:
enum StarRating {
VeryPoor = 1,
Poor,
Average,
Good,
Excellent
}
In this case, the first member will be assigned the value of 1. The subsequent choices will be auto-incremented from the previous choice. So Poor will have a rating of 2, an average of 3, and so on.
Choices in an enum do not always have numerical values, as you will see later. However, those with numerical values will behave like normal numbers.
console.log(typeof StarRating.Average);
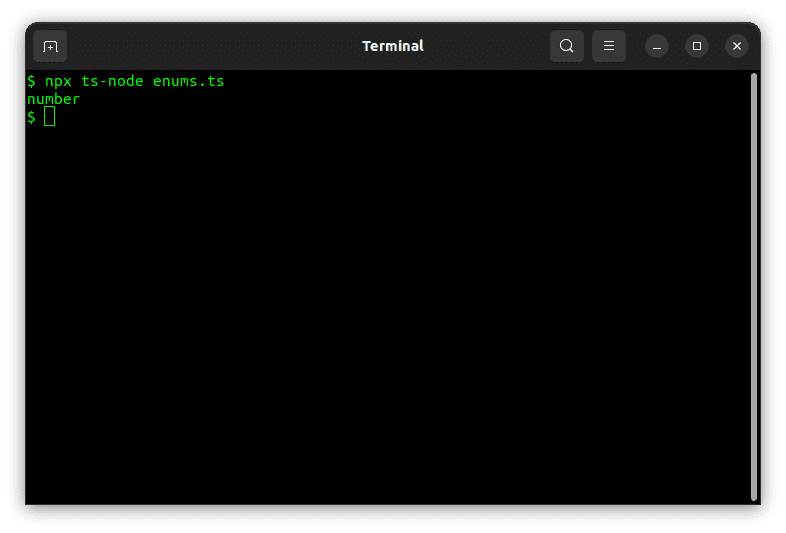
This will print "number"
. This means you can do all the number stuff, including making comparisons such as:
console.log(StarRating.Excellent > StarRating.Average)

This will print "true"
.
Using Strings as Custom Values
As mentioned earlier, enum choices can have string values instead of just numbers. Here’s how you would do that:
enum Choice {
RedPill = "Know Everything"
BluePill = "Know Nothing"
}
In this case, the enum choices will behave like the previous ones. However, because the values are strings, you can perform string operations, not number operations, like in the previous example.
Heterogenous Enums
You can also mix string and numerical values. This is discouraged and not very useful, but possible nonetheless.
enum Mixed {
First = "String"
Second = 2
}
Final Words
In this article, we covered what enums in TypeScript are and why they are important. Further, we covered the different kinds of values enum members can have.
Next, check out our article on Type vs. Interface in TypeScript.
-
Full stack web developer and technical writer. Currently learning AI.
-
Rashmi has over 7 years of expertise in content management, SEO, and data research, making her a highly experienced professional. She has a solid academic background and has done her bachelor’s and master’s degree in computer applications…. read more